Identify users
The mandatory user hash guarantees that users are who they claim to be. Otherwise, a user could manually run Javascript commands to impersonate another one and view their conversations.
You can configure Front Chat to accept both anonymous and identified users. You can also completely disable anonymous users to avoid any confusion.
The value of the email
field should be a valid email address. This value is used in Front to associate a new or existing contact with a conversation they have started via Chat:
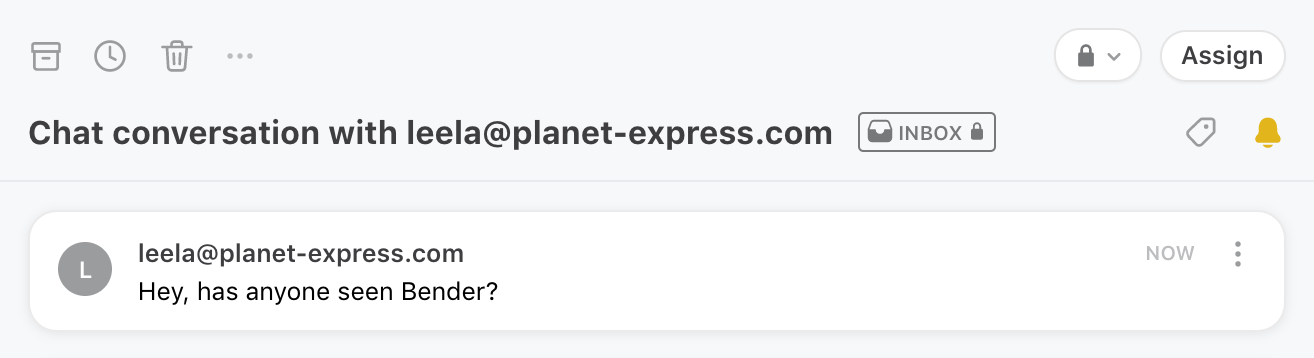
Computing the user hash
Front Chat uses a server-side generated HMAC (hash based message authentication code) with SHA-256. The identity verification will fail unless a user hash is provided.
// computing a user hash based on HMAC-SHA256
// Node.js example
const crypto = require('crypto');
const hmac = crypto.createHmac('sha256', verificationSecret);
const userHash = hmac.update(userEmail).digest('hex');
// computing a user hash based on HMAC-SHA256
// PHP example
$userHash = hash_hmac('sha256', $userEmail, $verificationSecret);
// computing a user hash based on HMAC-SHA256
// Ruby example
require 'openssl'
userHash = OpenSSL::HMAC.hexdigest(OpenSSL::Digest.new('sha256'), verificationSecret, userEmail)
import hashlib
import hmac
hmac.new(verification_secret, msg=user_email.encode('utf8'), digestmod=hashlib.sha256).hexdigest()
To compute a user hash, you will first need to retrieve your identity secret, which is available in your Front settings. Go to Settings > Inboxes > (Your chat inbox) > (Your chat channel) and expand the section "Verify logged-in user identity":
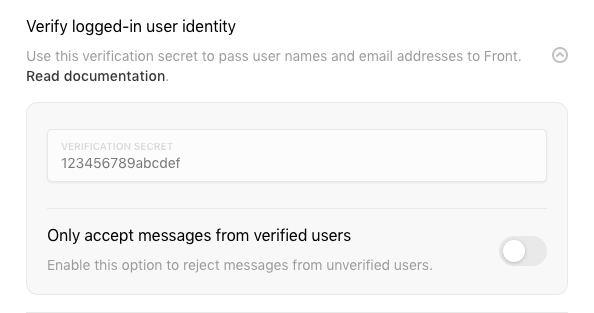
Important: the verification secret must remain private and must not appear in your frontend source code. The user hash must be computed in your backend, without disclosing the secret. The user hash must explicitly be generated from a user ID, if a user ID is being passed to the SDK.
Init with verified identity
You can optionally pass the identity of the user in the init
call.
FrontChat('init', {
useDefaultLauncher: false,
email: 'leela@planet-express.com',
userHash: '<generated using the verification secret>',
name: 'Turanga Leela',
customFields: { 'Packages delivered': 242 }
});
The identity of your user can be specified by using either the email
or userId
fields. In either case, the userHash
must be computed from the field that you plan to use as the identity. So if passing in userId
, you must compute userHash
from the userId
. If instead only an email
is passed in but you want to verify the user, then userHash
must be computed from the email
.
You may generate a userHash
based on the userId
and still pass an email
as part of a contact
object; this enables you to verify identity on an immutable field (userId
), and send the user's current email.
FrontChat('init', {
useDefaultLauncher: false,
name: 'Turanga Leela',
userHash: '<generated using the verification secret + userId>',
userId: 'cus_7ab2561',
contact: {
email: 'leela@planet-express.com',
customFields: { 'Packages delivered': 242 }
}
});
FrontChat('identity', object)
This method allows you to pass an identity object to Front in order for the user to be identified when they send messages. The object can take the following shape:
FrontChat('identity', {
email: 'leela@planet-express.com',
name: 'Turanga Leela',
userHash: '<generated using the verification secret>',
customFields: {
'Shipments delivered': 242,
Title: 'Parcel Captain',
'Is admin': true
}
});
All fields are optional (userHash
is only required when an email
or userId
is provided). The custom fields are defined by the custom fields for contacts that you have created in Front.
Note: if the user provides their name or email it will override what is provided in the identity information.
Updated about 1 month ago