Sample Applications
To demonstrate common Core API concepts, Front provides sample applications that help bootstrap your development.
Conversation/Message Import application
This example application demonstrates how to import conversations and messages to Front.
Conversations are the parent objects for the communications you have in Front inboxes. Each conversation contains messages that represent the actual communications between recipients.
For example, if [email protected]
sends an email to [email protected]
and [email protected]
, and then [email protected]
replies, you would have one conversation with two messages in it (one message from John, and one message from Gloria, all in the same conversation).
Source code
This project is available on GitHub.
Noteworthy concepts
This sample application demonstrates the following important Core API concepts:
- Importing messages—The application uses the buildRequest() function to map data from an example message file to interfaces that represent Front message types.
- Setting headers and attachments—The example application uses Needle to make the HTTP requests and demonstrates how to include headers and attachments.
- Rate limiting—Including rate limiting is extremely important to avoid missing data in your integrations, and this project demonstrates an example of how you can do so with the help of a utility function. Read more about rate limiting here.
Prerequisites
These applications use Node.js, Yarn, and TypeScript, but the concepts can be adapted to any framework. To run this application, however, you will need to install these frameworks.
Configuring the application
- Clone the repository from GitHub.
- In the project root, run
yarn install
to load the project dependencies. - In the
.env
file, add your API token to theAPI_KEY
variable.
How to generate an API tokenLearn about authentication here. For purposes of this sample application, you can create an API token to get up and running quickly. Just make sure it has a scope with permissions for the inboxes you want to import messages to. For production applications, you can consider implementing OAuth.
Keep the.env
file privateDo not commit the
.env
file to source code, as it can expose your private API key. This project is set up to not track changes to this file.
Running the demo message import
This application imports randomly generated messages into inboxes of your choice. By default, the example logic generates messages for two inboxes, [email protected]
and [email protected]
, and imports these conversations into two inboxes.
To run this example, complete the following steps:
- In src/inbox_mapping.json, update the two inbox values with the IDs for the inboxes you want to import messages to. You can use List inboxes to get a list of inbox IDs at your company. You can pick any two inboxes so long as your API token has access to them. You do not need to change the names of the inboxes in this file (
[email protected]
,[email protected]
). - (Optional) In src/examples.prepare.ts, change the
NUM_OF_MESSAGES
andNUM_OF_CONVERSATIONS
that the message generation script generates. You can also change the inbox handles increateRecipients
if you'd like, but make sure to update the names of the inboxes insrc/inbox_mapping.json
if you do (otherwise, there won't be any matches so the example logic won't import anything). - Run
yarn prepare
to generate the example messages. - Run
yarn import
to import the example messages to the inboxes you mapped insrc/inbox_mapping.json
.
The import process can take a few minutes (or longer) depending on the number of messages you import. The default number of 5 conversations and 25 messages shouldn't take longer than a minute to complete.
After the import completes, you should see the messages in the Front inboxes you mapped in src/inbox_mapping.json
. By default, the src/examples/prepare.ts
file generates messages with random creation dates, but all of them use the subject I am like the blue rose
. Searching for that phrase should make the messages easy to find.
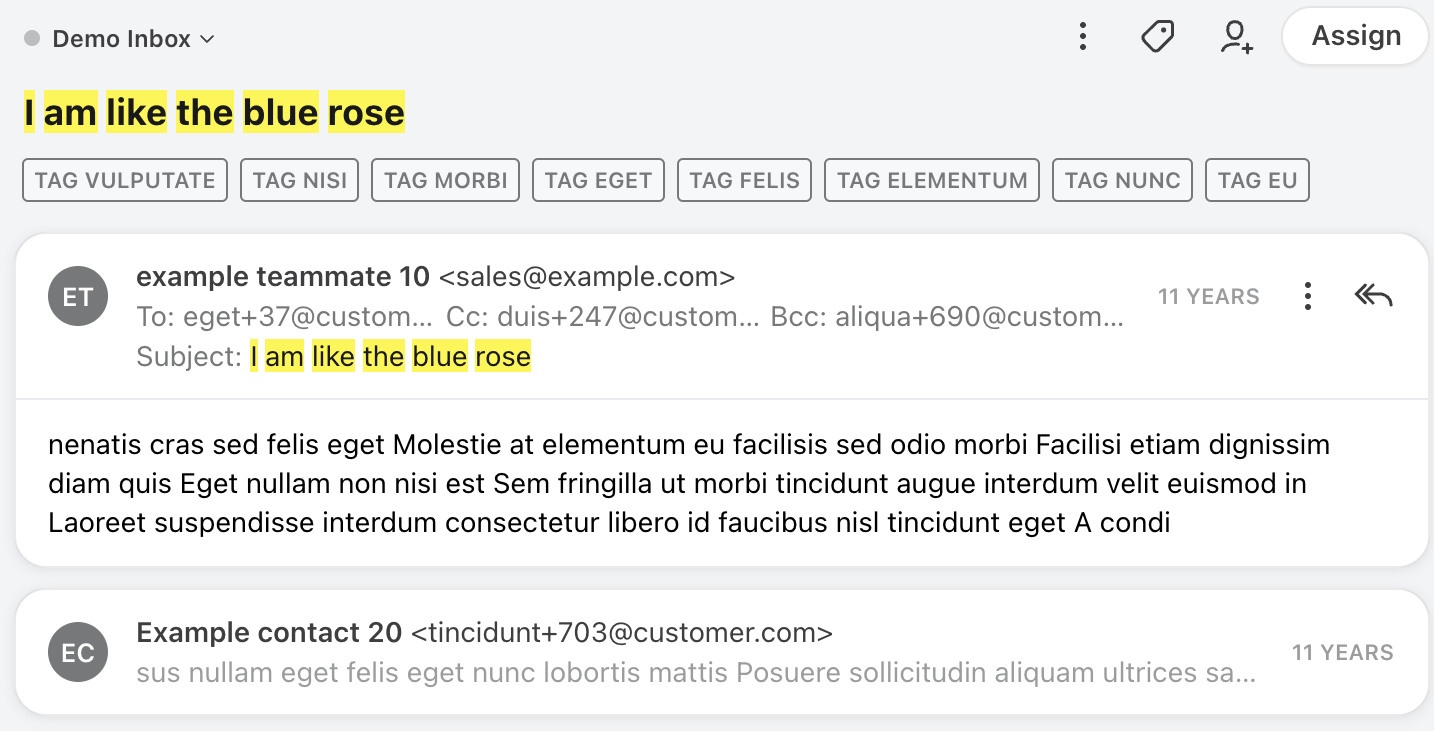
Adapting this sample application for production code
This sample application generates random messages and imports them into inboxes of your choosing. To adapt the code to a real-world use case:
-
Customize the
buildRequest()
function in src/index.ts to map messages from your external message system to the Front interface. You can customize the specifics of the mapping in this section of the function, which connects to the interfaces set up in the src/front_import.ts file. -
In the
main()
function in src/index.ts, import messages from your external message system rather than from the example file. -
In the
getInboxId()
function in src/index.ts, update how messages are assigned to inboxes during the import.The example code currently routes messages in two ways:
- For inbound messages, the email of the first recipient determines the inbox the message is imported to. In the real world, you would likely want to want to import the message to additional inboxes based on more detailed routing logic, such as the full list of participants or specific IDs in the subject or body text.
- For outgoing messages, the email of the sender determines the inbox that the messages are imported to. In the real world, you would likely want to want to import the message to additional inboxes based on more detailed routing logic, such as the full list of participants or specific IDs in the subject or body text.
- The mappings in the example code are based on on the src/inbox_mapping.json file, but you can build a more comprehensive mapping strategy for production code.
-
You can get rid of anything in the
examples
directory, as well as the import insrc/index.ts
, since these files are used only to generate example messages for this tutorial.
Conversation/Message Export application
This example application demonstrates how to export conversations and messages from Front. This is an ETL script, with Front serving as the extract point rather than the load point.
Conversations are the parent objects for the communications you have in Front inboxes. Each conversation contains messages that represent the actual communications between recipients.
For example, if [email protected]
sends an email to [email protected]
and [email protected]
, and then [email protected]
replies, you would have one conversation with two messages in it (one message from John, and one message from Gloria, all in the same conversation).
Source code
This project is available on GitHub.
Noteworthy concepts
This sample application demonstrates the following important Core API concepts:
- Searching for conversations—The application searches for conversations by status, exact phrase, and date filters. The exportSearchConversations function demonstrates how you might implement the Search conversations API endpoint.
- Handling pagination—The makePaginatedAPIRequest function demonstrates how to aggregate API resources returned by the Core API across multiple pages. You can see an example of a function that awaits the API to return all the inboxes for an account and utilizes the pagination function here.
- Exporting messages, comments, and attachments—The application demonstrates how to export conversations with all messages, comments, and attachments included.
- Typing responses from the Core API—The application contains non-exhaustive typing for responses from Front's API, which allows for easy casting in paginated responses.
- Rate limiting—Including rate limiting is extremely important to avoid missing data in your integrations, and this project demonstrates an example of how you can do so. Read more about rate limiting here.
Prerequisites
These applications use Node.js, Yarn, and TypeScript, but the concepts can be adapted to any framework. To run this application, however, you will need to install these frameworks.
Configuring the application
- Clone the repository from GitHub.
- In the project root, run
yarn install
to load the project dependencies. - In the
.env
file, add your API token to theAPI_KEY
variable.
How to generate an API tokenLearn about authentication here. For purposes of this sample application, you can create an API token to get up and running quickly. Just make sure it has a scope with permissions for the inboxes you want to import messages to. For production applications, you can consider implementing OAuth.
Keep the.env
file privateDo not commit the
.env
file to source code, as it can expose your private API key. This project is set up to not track changes to this file.
Running the demo message export
This application searches for conversations that match the criteria specified in the configuration file. The application searches through all the inboxes that your API token has access to. For each conversation that matches, the export application will generate JSON files that contain the conversation details, including the details of the messages, comments, and attachments of the conversation (depending on which options you enabled in the configuration).
To run this example, complete the following steps:
- In src/index.ts, choose:
- Whether to include messages, comments, and attachments in the options.
- How to search for conversations.
- The first argument is a phrase the conversation should contain. Use double quotes if you want an exact match, or omit the double quotes if you desire partial matching.
- The second argument is a
before
,after
, orduring
Unix timestamp for when the first message in the conversation was created. Read more about these filters in our Search topic. - The third argument is the conversation status. Read more about the
is
status filter in our our Search topic.
- If you want to search for all messages in an inbox, you can adapt the code to
FrontExport.exportSearchConversations(*inbox*, options)
. - If you want to search for all messages across all inboxes, uncomment this section and comment out this section.
- By default, the export application includes all messages, comments, and attachments, and searches for conversations that contain the exact phrase "cup of coffee", were created after September 13th, 2020, and have an Open status.
The export process can take a while to completeThe export process can take a lengthy time to complete, depending on how many conversations match your search. We recommend starting with a conversation search that will return limited results so that you can gauge the time it might take for a full export in a real-world scenario.
- Run
yarn start
to run the export.
After the export completes, the application generates an export
directory that contains JSON files for each conversation and its associated messages that matched your search.
Adapting this sample application for production code
This sample application generates JSON files for conversations that match a search query. To adapt this application for production code, you should consider the following items:
- Customize the conversation search for the real-world use case you are implementing. You can augment the exportSearchConversations function to include more filters in the query arguments provided by the sample application.
- Define how you would like to load the extracted data in the src/helpers.ts file. This sample application only writes the data to an
export
folder. - Consider filtering which inboxes the export applies to. The sample application currently lists all inboxes the API token has access to.
Updated 7 months ago